In this blog post we will see how to use Python and REST APIs with NSX manager.
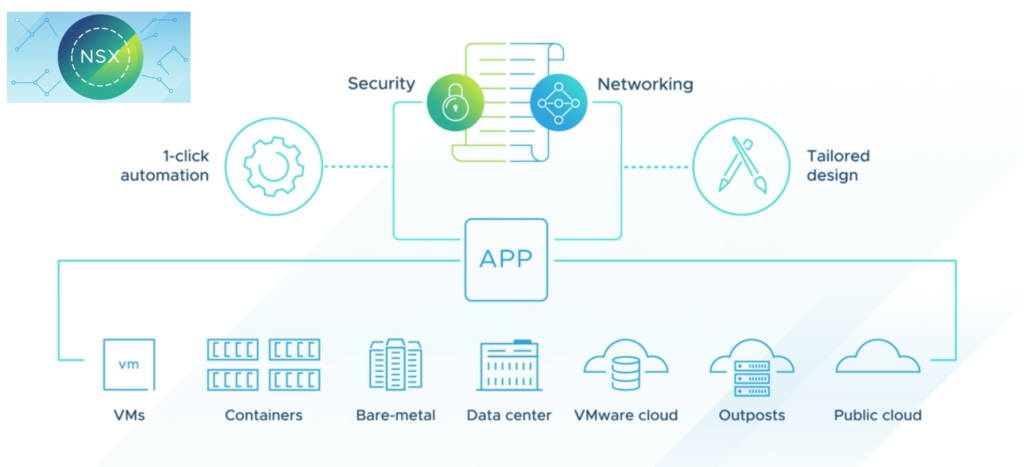
NSX-T manager provides programmatic access to automate NSX-T Data Center using REST API. In this blog post we also see how can we utilize Python SDK for NSX-T.
As per API guide, NSX-T APIs follow REST architecture using JSON object encoding. Clients interact with the API using RESTful web service calls over HTTPS protocol. We can authenticate API calls using HTTP Basic Authentication, Session-Based Authentication and X.509 certificate and Principal Identity.
Using Python requests library
Let’s write a simple code to get transport zone and transport node information from NSX-T manager. Username and password should be entered using Base64 encoding in the following format ‘Username:Password’. This is a very basic example and is great for understanding, the same code can be optimized for better re-usability.
import requests
url_transport_zones = "https://sa-nsxmgr-01.clitoapi.local/api/v1/transport-zones"
url_transport_nodes = "https://sa-nsxmgr-01.clitoapi.local/api/v1/transport-nodes"
headers = {
'Authorization': "Basic VVNFUjpQQVNTV09SRA==",
}
transport_zones = requests.request("GET", url_transport_zones, headers=headers, verify=False)
transport_node = requests.request("GET", url_transport_nodes, headers=headers, verify=False)
print("\n\n ---- Transport Zones ---- \n\n ")
print(transport_zones.text)
print("\n ---- Transport Nodes ---- \n")
print(transport_node.text)
The above code only utilizes requests library and REST APIs to interact with NSX manager.
Here is an example of how to create a transport zone in NSX-T manager.
import requests
url = "https://sa-nsxmgr-01.clitoapi.local/api/v1/transport-zones"
payload="{\r\n \"display_name\":\"tz2\",\r\n \"description\":\"Transport Zone 1\",\r\n \"transport_type\":\"OVERLAY\"\r\n}"
headers = {
'Authorization': 'Basic VVNFUjpQQVNTV09SRA==',
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload, verify=False)
print(response.text)
Using NSX SDK for Python
VMware provides NSX-T for Python SDK (Software Development Kit) that can used for automating NSX-T configuration and management. It includes very useful python libraries for accessing NSX-T features using REST API. You can download SDK from VMware Developer page. Here is direct link to SDK, you can download a version depending upon NSX-T manager version. SDK is available under Drivers & Tools section.
https://developer.vmware.com/web/sdk/3.2.1/nsx-t-python
In my setup I installed the following whl files. You can use pip to install the files.
pip install vapi_runtime-2.29.0-py2.py3-none-any.whl
pip install vapi_common-2.29.0-py2.py3-none-any.whl
pip install vapi_common_client-2.29.0-py2.py3-none-any.whl
pip install nsx_python_sdk-3.2.0.1.0-py2.py3-none-any.whl
pip install nsx_policy_python_sdk-3.2.0.1.0-py2.py3-none-any.whl
You can also create a python virtual environment if you need but we will not cover virtual environment in this blog post.
Once these packages are installed we are ready to write first script using NSX SDK for Python. We will get list of transport zones from NSX-T manager.
import pprint
import requests
from com.vmware.nsx_client import TransportZones
from vmware.vapi.bindings.struct import PrettyPrinter
from vmware.vapi.lib import connect
from vmware.vapi.security.user_password import \
create_user_password_security_context
from vmware.vapi.stdlib.client.factories import StubConfigurationFactory
def main():
# Create a session using the requests library.
session = requests.session()
# If manager is running self-signed certificate set verify to False.
session.verify = False
# Set up the API connector.
nsx_url = 'https://%s:%s' % ("sa-nsxmgr-01.clitoapi.local", 443)
connector = connect.get_requests_connector(
session=session, msg_protocol='rest', url=nsx_url)
stub_config = StubConfigurationFactory.new_std_configuration(connector)
# Enter username and password for NSX-T manager.
security_context = create_user_password_security_context(
"admin", "password1!")
connector.set_security_context(security_context)
# Get list of transport zones
transportzones_svc = TransportZones(stub_config)
tzs = transportzones_svc.list()
# Create a pretty printer to make the output look nice.
pretty_print = PrettyPrinter()
pretty_print.pprint(tzs)
if __name__ == "__main__":
main()
If we check installed python packages we can find StubConfigurationFactory class, which is used for creating configuration objects.
class StubConfigurationFactory(object):
"""
Factory class for creating stub configuration objects
"""
In TransportZones class you can see ‘list’ function used to get transport zones as output.
class TransportZones(VapiInterface):
"""
"""
LIST_TRANSPORT_TYPE_OVERLAY = "OVERLAY"
"""
Possible value for ``transportType`` of method :func:`TransportZones.list`.
"""
LIST_TRANSPORT_TYPE_VLAN = "VLAN"
"""
Possible value for ``transportType`` of method :func:`TransportZones.list`.
"""
_VAPI_SERVICE_ID = 'com.vmware.nsx.transport_zones'
"""
def list(self,
cursor=None,
display_name=None,
include_system_owned=None,
included_fields=None,
is_default=None,
page_size=None,
sort_ascending=None,
sort_by=None,
transport_type=None,
uplink_teaming_policy_name=None,
):
You can read more reference examples here, https://blogs.vmware.com/networkvirtualization/2018/01/nsx-t-openapi-sdks.html/.